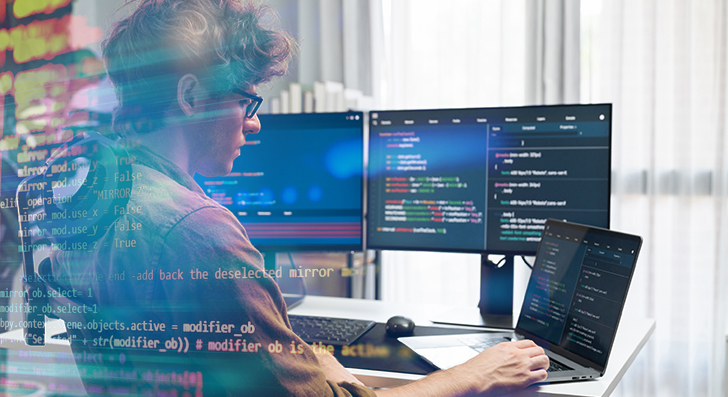
Scalability indicates your application can deal with advancement—additional end users, a lot more data, and more targeted visitors—devoid of breaking. Like a developer, developing with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the start. A lot of applications fall short when they increase fast due to the fact the original layout can’t handle the extra load. As being a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases where every little thing is tightly related. Rather, use modular layout or microservices. These styles crack your app into more compact, unbiased elements. Just about every module or service can scale on its own with no influencing The complete method.
Also, think of your databases from working day one particular. Will it have to have to handle a million consumers or maybe 100? Pick the ideal type—relational or NoSQL—according to how your details will grow. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them still.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only will work less than present-day disorders. Think about what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design styles that aid scaling, like information queues or celebration-pushed programs. These support your app manage additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you happen to be lowering potential complications. A properly-planned system is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Databases
Selecting the right databases can be a crucial part of setting up scalable apps. Not all databases are constructed the same, and utilizing the Completely wrong one can slow you down or maybe result in failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. They also guidance scaling methods like study replicas, indexing, and partitioning to manage much more targeted visitors and info.
If your knowledge is a lot more flexible—like person activity logs, merchandise catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and produce styles. Have you been executing plenty of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases which can deal with substantial produce throughput, or maybe function-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to think ahead. You may not need to have Highly developed scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your entry designs. And generally watch databases effectiveness when you improve.
In short, the proper database depends on your app’s composition, velocity desires, And just how you be expecting it to increase. Just take time to choose correctly—it’ll help save a great deal of difficulties later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately penned code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Commence by creating clean, very simple code. Keep away from repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a simple just one operates. Keep your features brief, concentrated, and simple to test. Use profiling tools to search out bottlenecks—areas where your code can take as well extensive to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These frequently gradual issues down much more than the code by itself. Be certain Each and every question only asks for the data you really need. Prevent Choose *, which fetches anything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout big tables.
In case you notice precisely the same details becoming asked for repeatedly, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations once you can. In place of updating a row one by one, update them in groups. This cuts down on overhead and can make your application additional efficient.
Remember to check with huge datasets. Code and queries that get the job done great with a hundred records may crash after they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your application remain clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and more visitors. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. In lieu of a person server executing every one of the operate, the load balancer routes consumers to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it may be reused speedily. When consumers ask for precisely the same details again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces database load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter generally. And usually be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. With each other, they assist your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Instruments
To make scalable applications, you may need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you adaptability, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just some clicks or mechanically working with vehicle-scaling. When website traffic drops, you could scale down to economize.
These platforms also present services like managed databases, storage, load balancing, and security applications. You can deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it should run—code, libraries, configurations—into one device. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of your respective app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into products and services. It is possible to update or scale components independently, and that is great for general performance and dependability.
In short, employing cloud and container resources suggests you are able to scale speedy, deploy very easily, and recover promptly when issues transpire. If you'd like your application to grow with no boundaries, get started making use of these instruments early. They save time, lessen risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t watch your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking primary metrics like CPU use, memory, disk House, and response time. These inform you how your servers and expert services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this check here facts.
Don’t just observe your servers—observe your application too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Build alerts for significant challenges. Such as, If the reaction time goes previously mentioned a limit or even a services goes down, you need to get notified instantly. This helps you fix challenges rapid, generally ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right applications in position, you continue to be on top of things.
In short, checking assists you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the appropriate tools, it is possible to build apps that improve smoothly with no breaking under pressure. Start off compact, Feel major, and build wise.